Posted by Xiaodao Wu – Developer Relations Engineer
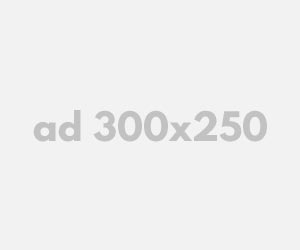
Jetpack WindowManager keeps getting better. WindowManager gives you tools to build adaptive apps that work seamlessly across all kinds of large screen devices. Version 1.4, which is stable now, introduces new features that make multi-window experiences even more powerful and flexible. While Jetpack Compose is still the best way to create app layouts for different screen sizes, 1.4 makes some big improvements to activity embedding, including activity stack spinning, pane expansion, and dialog full-screen dim. Multi-activity apps can easily take advantage of all these great features.
WindowManager 1.4 introduces a range of enhancements. Here are some of the highlights.
WindowSizeClass
We’ve updated the WindowSizeClass API to support custom values. We changed the API shape to make it easy and extensible to support custom values and add new values in the future. The high level changes are as follows:
Opened the constructor to take in minWidthDp and minHeightDp parameters so you can create your own window size classes
Added convenience methods for checking breakpoint validity
Deprecated WindowWidthSizeClass and WindowHeightSizeClass in favor of WindowSizeClass#isWidthAtLeastBreakpoint() and WindowSizeClass#isHeightAtLeastBreakpoint() respectively
Here’s a migration example:
// old
val sizeClass = WindowSizeClass.compute(widthDp, heightDp)
when (sizeClass.widthSizeClass) {
COMPACT -> doCompact()
MEDIUM -> doMedium()
EXPANDED -> doExpanded()
else -> doDefault()
}
// new
val sizeClass = WindowSizeClass.BREAKPOINTS_V1
.computeWindowSizeClass(widthDp, heightDp)
when {
sizeClass.isWidthAtLeastBreakpoint(WIDTH_DP_EXPANDED_LOWER_BOUND) -> {
doExpanded()
}
sizeClass.isWidthAtLeastBreakpoint(WIDTH_DP_MEDIUM_LOWER_BOUND) -> {
doMedium()
}
else -> {
doCompact()
}
}
Some things to note in the new API:
The order of the when branches should go from largest to smallest to support custom values from developers or new values in the future
The default branch should be treated as the smallest window size class
Activity embedding
Activity stack pinning
Activity stack pinning provides a way to keep an activity stack always on screen, no matter what else is happening in your app. This new feature lets you pin an activity stack to a specific window, so the top activity stays visible even when the user navigates to other parts of the app in a different window. This is perfect for things like live chats or video players that you want to keep on screen while users explore other content.
private fun pinActivityStackExample(taskId: Int) {
val splitAttributes: SplitAttributes = SplitAttributes.Builder()
.setSplitType(SplitAttributes.SplitType.ratio(0.66f))
.setLayoutDirection(SplitAttributes.LayoutDirection.LEFT_TO_RIGHT)
.build()
val pinSplitRule = SplitPinRule.Builder()
.setDefaultSplitAttributes(splitAttributes)
.build()
SplitController.getInstance(applicationContext).pinTopActivityStack(taskId, pinSplitRule)
}
Pane expansion
The new pane expansion feature, also known as interactive divider, lets you create a visual separation between two activities in split-screen mode. You can make the pane divider draggable so users can resize the panes – and the activities in the panes – on the fly. This gives users control over how they want to view the app’s content.
val splitAttributesBuilder: SplitAttributes.Builder = SplitAttributes.Builder()
.setSplitType(SplitAttributes.SplitType.ratio(0.33f))
.setLayoutDirection(SplitAttributes.LayoutDirection.LEFT_TO_RIGHT)
if (WindowSdkExtensions.getInstance().extensionVersion >= 6) {
splitAttributesBuilder.setDividerAttributes(
DividerAttributes.DraggableDividerAttributes.Builder()
.setColor(getColor(context, R.color.divider_color))
.setWidthDp(4)
.setDragRange(
DividerAttributes.DragRange.DRAG_RANGE_SYSTEM_DEFAULT)
.build()
)
}
val splitAttributes: SplitAttributes = splitAttributesBuilder.build()
Dialog full-screen dim
WindowManager 1.4 gives you more control over how dialogs dim the background. With dialog full-screen dim, you can choose to dim just the container where the dialog appears or the entire task window for a unified UI experience. The entire app window dims by default when a dialog opens (see EmbeddingConfiguration.DimAreaBehavior.ON_TASK).To dim only the container of the activity that opened the dialog, use EmbeddingConfiguration.DimAreaBehavior.ON_ACTIVITY_STACK. This gives you more flexibility in designing dialogs and makes for a smoother, more coherent user experience. Temu is among the first developers to integrate this feature, the full-screen dialog dim has reduced screen invalid touches by about 5%.
Customised shopping cart reminder with dialog full-screen dim in Temu.
Enhanced posture support
WindowManager 1.4 makes building apps that work flawlessly on foldables straightforward by providing more information about the physical capabilities of the device. The new WindowInfoTracker#supportedPostures API lets you know if a device supports tabletop mode, so you can optimize your app’s layout and features accordingly.
val currentSdkVersion = WindowSdkExtensions.getInstance().extensionVersion
val message =
if (currentSdkVersion >= 6) {
val supportedPostures = WindowInfoTracker.getOrCreate(LocalContext.current).supportedPostures
buildString {
append(supportedPostures.isNotEmpty())
if (supportedPostures.isNotEmpty()) {
append(” “)
append(
supportedPostures.joinToString(
separator = “,”, prefix = “(“, postfix = “)”))
}
}
} else {
“N/A (WindowSDK version 6 is needed, current version is $currentSdkVersion)”
}
Other API changes
WindowManager 1.4 includes several API changes and additions to support the new features. Notable changes include:
Stable and no longer experimental APIs:
ActivityEmbeddingController#invalidateVisibleActivityStacks
ActivityEmbeddingController#getActivityStack
SplitController#updateSplitAttributes
API added to set activity embedding animation background:
SplitAttributes.Builder#setAnimationParams
API to get updated WindowMetrics information:
ActivityEmbeddingController#embeddedActivityWindowInfo
API to finish all activities in an activity stack:
ActivityEmbeddingController#finishActivityStack
How to get started
To start using Jetpack WindowManager 1.4 in your Android projects, update your app dependencies in build.gradle.kts to the latest stable version:
dependencies {
implementation(“androidx.window:window:1.4.0”)
// or, if you’re using the WindowManager testing library:
testImplementation(“androidx.window:window-testing:1.4.0”)
}
Happy coding!
GIPHY App Key not set. Please check settings