Posted by Thomas Ezan – Sr. Developer Relation Engineer (@lethargicpanda)
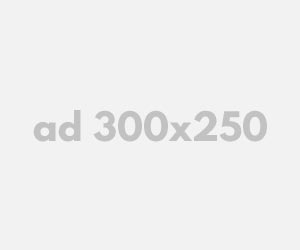
Gemini can help you build and launch new user features that will boost engagement and create personalized experiences for your users.
The Vertex AI in Firebase SDK lets you access Google’s Gemini Cloud models (like Gemini 1.5 Flash and Gemini 1.5 Pro) and add GenAI capabilities to your Android app. It became generally available last October which means it’s now ready for production and it is already used by many apps in Google Play.
Here are tips for a successful deployment to production.
Implement App Check to prevent API abuse
When using the Vertex AI in Firebase API it is crucial to implement robust security measures to prevent unauthorized access and misuse.
Firebase App Check helps protect backend resources (like Vertex AI in Firebase, Cloud Functions for Firebase, or even your own custom backend) from abuse. It does this by attesting that incoming traffic is coming from your authentic app running on an authentic and untampered Android device.
Firebase App Check ensures that only legitimate
users access your backend resources
To get started, add Firebase to your Android project and enable the Play Integrity API for your app in the Google Play console. Back in the Firebase console, go to the App Check section of your Firebase project to register your app by providing its SHA-256 fingerprint.
Then, update your Android project’s Gradle dependencies with the App Check library for Android:
dependencies {
// BoM for the Firebase platform
implementation(platform(“com.google.firebase:firebase-bom:33.7.0”))
// Dependency for App Check
implementation(“com.google.firebase:firebase-appcheck-playintegrity”)
}
Finally, in your Kotlin code, initialize App Check before using any other Firebase SDK:
Firebase.initialize(context)
Firebase.appCheck.installAppCheckProviderFactory(
PlayIntegrityAppCheckProviderFactory.getInstance(),
)
To enhance the security of your generative AI feature, you should implement and enforce App Check before releasing your app to production. Additionally, if your app utilizes other Firebase services like Firebase Authentication, Firestore, or Cloud Functions, App Check provides an extra layer of protection for those resources as well.
Once App Check is enforced, you’ll be able to monitor your app’s requests in the Firebase console.
App Check metrics page in the Firebase console
You can learn more about App Check on Android in the Firebase documentation.
Use Remote Config for server-controlled configuration
The generative AI landscape evolves quickly. Every few months, new Gemini model iterations become available and some models are removed. See the Vertex AI in Firebase Gemini models page for details.
Because of this, instead of hardcoding the model name in your app, we recommend using a server-controlled variable using Firebase Remote Config. This allows you to dynamically update the model your app uses without having to deploy a new version of your app or require your users to pick up a new version.
You define parameters that you want to control (like model name) using the Firebase console. Then, you add these parameters into your app, along with default “fallback” values for each parameter. Back in the Firebase console, you can change the value of these parameters at any time. Your app will automatically fetch the new value.
Here’s how to implement Remote Config in your app:
// Initialize the remote configuration by defining the refresh time
val remoteConfig: FirebaseRemoteConfig = Firebase.remoteConfig
val configSettings = remoteConfigSettings {
minimumFetchIntervalInSeconds = 3600
}
remoteConfig.setConfigSettingsAsync(configSettings)
// Set default values defined in your app resources
remoteConfig.setDefaultsAsync(R.xml.remote_config_defaults)
// Load the model name
val modelName = remoteConfig.getString(“model_name”)
Read more about using Remote Config with Vertex AI in Firebase.
Gather user feedback to evaluate impact
As you roll out your AI-enabled feature to production, it’s critical to build feedback mechanisms into your product and allow users to easily signal whether the AI output was helpful, accurate, or relevant. For example, you can incorporate interactive elements such as thumb-up and thumb-down buttons and detailed feedback forms within the user interface. The Material Icons in Compose package provides ready to use icons to help you implement it.
You can easily track the user interaction with these elements as custom analytics events by using Google Analytics logEvent() function:
Row {
Button (
onClick = {
firebaseAnalytics.logEvent(“model_response_feedback”) {
param(“feedback”, “thumb_up”)
}
}
) {
Icon(Icons.Default.ThumbUp, contentDescription = “Thumb up”)
},
Button (
onClick = {
firebaseAnalytics.logEvent(“model_response_feedback”) {
param(“feedback”, “thumb_down”)
}
}
) {
Icon(Icons.Default.ThumbDown, contentDescription = “Thumb down”)
}
}
Learn more about Google Analytics and its event logging capabilities in the Firebase documentation.
User privacy and responsible AI
When you use Vertex AI in Firebase for inference, you have the guarantee that the data sent to Google won’t be used by Google to train AI models (see Vertex AI documentation for details).
It’s also important to be transparent with your users when they’re engaging with generative AI technology. You should highlight the possibility of unexpected model behavior.
Finally, users should have control within your app over how their activity related to AI model interactions is stored and deleted.
You can learn more about how Google is approaching Generative AI responsibly in the Google Cloud documentation.
GIPHY App Key not set. Please check settings